9.1. RS232 communications example
RS232 Communications Unit
//------------------------------------------------ ---------------------------
# Include
# Pragma hdrstop
# Include "RS232Unit.h"
//------------------------------------------------ ---------------------------
# Pragma package (smart_init)
# Pragma link "Comm"
# Pragma resource "*. dfm"
TForm1 * Form1;
//------------------------------------------------ ---------------------------
__fastcall TForm1:: TForm1 (TComponent * Owner)
: TForm (Owner)
{
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: FormCreate (TObject * Sender)
{
/ / Set the default communications
ComboBox1-> ItemIndex = br9600; / / Bits per second
/ * 0: br110
1: BR300
2: br600
3: br1200
4: br2400
5: br4800
6: br9600
7: br14400
8: br19200
9: br38400
10: br56000
11: br57600
12: br115200 * /
ComboBox2-> ItemIndex = DA8 / / Data Bits
/ * 0: da4
1: Qa5
2: DA6
3: DA7
4: DA8 * /
ComboBox3-> ItemIndex = Panone / / Parity
/ * 0: Panone
1: paOdd (Par)
2: paEven (odd)
3: paMark (Brand)
4: PASPAC (Space) * /
ComboBox4-> ItemIndex = SB10; / / Stop Bits
/ * 0: SB10
1: SB 15
2: SB20 * /
ComboBox5-> ItemIndex = fcNone / / Flow Control
/ * 0: fcNone
1: fcCTS
2: fcDTR
3: fcSottware
4: fcDefault * /
ComboBox6-> ItemIndex = 0, / / Port
}
//------------------------------------------------ ---------------------------
/ / Green Check OFF ON clLime clGreen
/ / Blue Check OFF ON 0x00FF8000 0x00804000
void __fastcall TForm1:: Comm1RxChar (TObject * Sender, DWORD Count)
{
int state;
int NumRec;
Dat String;
BufRec char [101];
Shape3-> Brush-> Color = clLime;
NumRec = comm1-> InQueCount (), / / Number of characters received
State = comm1-> Read (BufRec, Count) / / Read Buffer
if (state ==- 1)
{
ShowMessage ("Error in receiving");
}
else
{
for (int n = 0, n <NumRec, n + +)
{
if (! CheckBox1-> Checked)
{
Dat = dat + IntToStr (BufRec [n ])+',';
}
else
{
Dat = dat + BufRec [n];
}
}
Edit2-> Text = Edit2-> Text + Dat;
}
Shape2-> Brush-> Color = 0x00FF8000;
Timer1-> Enabled = true;
}
//------------------------------------------------ ---------------------------
void TForm1:: EstadoLineas ()
{
if (comm1-> CTS)
{
Shape8-> Brush-> Color = 0x00FF8000;
}
else
{
Shape8-> Brush-> Color = 0x00804000;
}
if (comm1-> DSR)
{
Shape6-> Brush-> Color = 0x00FF8000;
}
else
{
Shape6-> Brush-> Color = 0x00804000;
}
if (comm1-> RING)
{
Shape9-> Brush-> Color = 0x00FF8000;
}
else
{
Shape9-> Brush-> Color = 0x00804000;
}
if (comm1-> RLSD)
{
Shape1-> Brush-> Color = 0x00FF8000;
}
else
{
Shape1-> Brush-> Color = 0x00804000;
}
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Comm1Cts (TObject * Sender)
{
/ / Check the status of the CTS and DSR LINES
EstadoLineas ();
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Comm1Dsr (TObject * Sender)
{
/ / Check the status of the CTS and DSR LINES
EstadoLineas ();
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: BitBtn1Click (TObject * Sender)
{
if (! comm1-> Enabled ())
{
/ / Set parameters
Comm1-> DeviceName = ComboBox6-> Items-> Strings [ComboBox6-> ItemIndex] / / Port
Comm1-> baudrate = ComboBox1-> ItemIndex, / / Bits per second
Comm1-> DataBits = ComboBox2-> ItemIndex / / Data Bits
Comm1-> Parity = ComboBox3-> ItemIndex / / Parity
Comm1-> StopBits = ComboBox4-> ItemIndex / / Stop Bits
Comm1-> FlowControl = ComboBox5-> ItemIndex / / Flow Control
/ / Open communication port
Comm1-> Open ();
Comm1-> SetRTSState (true) / / Enable RTS
Shape7-> Brush-> Color = clLime;
Comm1-> SetDTRState (true) / / Enable DTR line
Shape4-> Brush-> Color = clLime;
/ / Toggle the status indicators open port
Label7-> Caption = "Open Port";
Shape10-> Brush-> Color = clLime;
/ / Check the status of the CTS and DSR LINES
EstadoLineas ();
}
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: BitBtn2Click (TObject * Sender)
{
if (comm1-> Enabled ())
{
/ / Close the communication port
Comm1-> Close ();
/ / Toggle the status indicators open port
Label7-> Caption = "Port Closed";
Shape10-> Brush-> Color = clRed;
Shape7-> Brush-> Color = clGreen;
Shape4-> Brush-> Color = clGreen;
/ / Check the status of the CTS and DSR LINES
EstadoLineas ();
}
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: BitBtn4Click (TObject * Sender)
{
Edit2-> Text = "";
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: BitBtn3Click (TObject * Sender)
{
Edit1-> Text = "";
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Comm1Ring (TObject * Sender)
{
/ / Check the status of the CTS and DSR LINES
EstadoLineas ();
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Comm1Rlsd (TObject * Sender)
{
/ / Check the status of the CTS and DSR LINES
EstadoLineas ();
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Edit1KeyPress (TObject * Sender, char & Key)
{
char dat [2];
Dat [0] = Key;
Comm1-> Write (dat, 1);
Shape3-> Brush-> Color = clLime;
Timer2-> Enabled = true;
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Timer1Timer (TObject * Sender)
{
Timer1-> Enabled = false;
Shape2-> Brush-> Color = 0x00804000;
}
//------------------------------------------------ ---------------------------
void __fastcall TForm1:: Timer2Timer (TObject * Sender)
{
Timer2-> Enabled = false;
Shape3-> Brush-> Color = clGreen;
}
//------------------------------------------------ ---------------------------
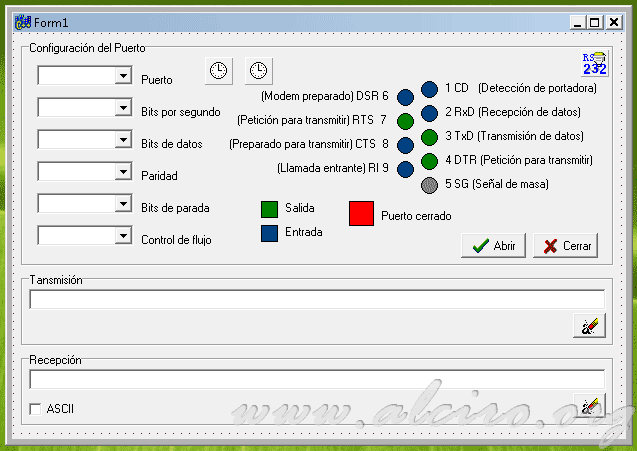
Example test program RS-232 communications